You can stop looking for guide on Powershell replace string in file, cause I have the best guide for you that will help you.
Powershell Replace String In File
There are two methods on replacing string in file.
In this example, I will use text file from location “C:\temp\GeekZag.txt“, which has content as below:
GeekZag website is awesome!
GeekZag content is powerful!
All String
To replace all string in a text file, it involves three(3) steps only which are get the file content, replace the string and save the file. I will guide you on how to construct the script/command so you will have clear picture on how it works.
Firstly, before anything, you must get the file content. Just need to use command Get-Content -path “File Path”. The command will be as below:
Get-Content -path “C:\temp\GeekZag.txt”
Then use command (Get-Content -Path “File Path”) -replace “from this”, “to this” as below to replace string is to really:
(Get-Content -path “C:\temp\GeekZag.txt”) -replace “is”, “really”
Finally, save the content of file by adding command Set-Content -Path “File Path”
(Get-Content -path “C:\temp\GeekZag.txt”) -replace “is”, “really” | Set-Content -Path “C:\temp\GeekZag.txt”
(Note: Command above is in single line. Ensure, the file is not open. Otherwise, you will get error saying the file is currently in use.)
Below is the result when running the command:
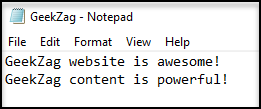
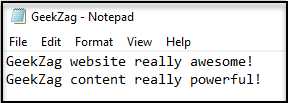
Conditional Filtering
You may not want to replace all string in a file, but only replace the string that meet some criteria. Don’t worry, you can use match string condition. This can be achieved easily using if conditional statement.
I will guide you on how to construct the script/command so you will have clear picture on how it works.
First of all, you need to read the text file’s content. It does not same as previous method. The command will be as below:
$stream_reader = New-Object System.IO.StreamReader{C:\temp\GeekZag.txt}
$line_number = 1
while (($current_line =$stream_reader.ReadLine()) -ne $null)
{
Write-Host “$line_number $current_line”
$line_number++
}
Explanation:
$stream_reader = New-Object System.IO.StreamReader{File Path}, to read the file.
$line_number = 1, as a counter for example
while (($current_line =$stream_reader.ReadLine()) -ne $null), read line by line while line is not empty
Write-Host “$line_number $current_line”, to result the command on Powershell windows.
Then, add if with -match string condition. In this example, we will try to find line with string ‘awesome’. You can always replace ‘awesome’ string to any words that fit your requirement.
if ($current_line -match “awesome”) {}
Then, inside the if statement, add the replace command as below:
if ($current_line -match “awesome”)
{
$newWord = $current_line -replace “is”, “really”
Write-Host “$line_number $newWord”
$line_number++
}
Below is the full Powershell script with conditional filtering.
$stream_reader = New-Object System.IO.StreamReader{C:\temp\GeekZag.txt}
$line_number = 1
while (($current_line =$stream_reader.ReadLine()) -ne $null)
{
if ($current_line -match “awesome”)
{
$newWord = $current_line -replace “is”, “really”
Write-Host “$line_number $newWord”
$line_number++
}
}
But, this method can’t use Set-Content to save it into a file. You need to save it into a new file.
To do so, you just need to use command as below:
$stream_reader = New-Object System.IO.StreamReader{C:\temp\GeekZag.txt}
$line_number = 1
while (($current_line =$stream_reader.ReadLine()) -ne $null)
{
if ($current_line -match “awesome”)
{
$newWord = $current_line -replace “is”, “really”
Write-Host “$line_number $newWord”
Add-content -path “C:\Temp\GeekZag(NEW).txt” -value $newWord
$line_number++
}
else
{
Write-Host “$line_number $current_line”
Add-content -path “C:\Temp\GeekZag(NEW).txt” -value $current_line
$line_number++
}}
Not that the changes I made that in bold. I just add the match condition into a new file “GeekZag(NEW).txt” and else condition.
If the condition is not match, it will add the original line-content into the new file.
Below is the output of the command:
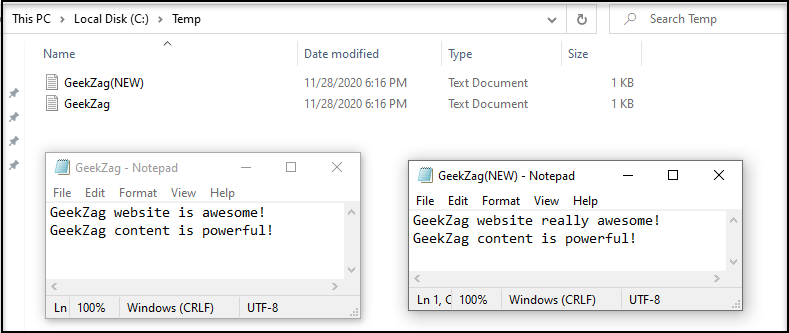
Thanks for reading this article, I hope you will find it helpful.
You may interest to read about Powershell Check Windows Service Status (Full Guides And Examples).